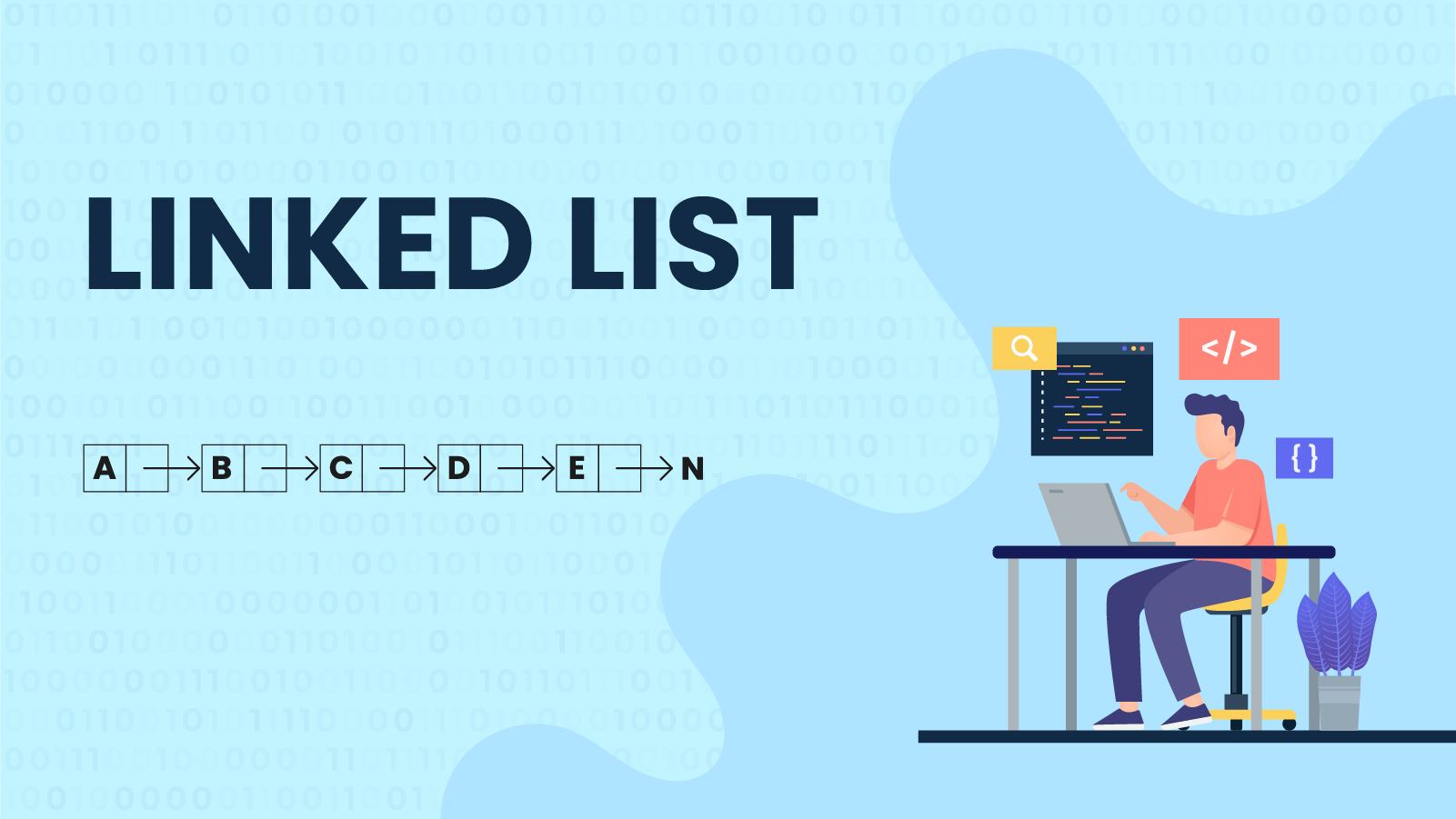
Here is a list of articles related to LINKED LIST DATA STRUCTURE
SINGLY LINKED LIST
- A Brief Introduction to Linked Lists
- Types of Linked list
- Advantage and Disadvantage of Linked List Over Array
- Advantages and Disadvantages of Linked List
- Applications of Linked List Data Structure
- Linked List – Inserting a node
- Why is Quicksort preferred for Arrays and Merge Sort for Linked Lists?
- Generic Linked List in C
- Linked List in C++
- Implementing a Linked List in Java using Class
- Java.util.LinkedList.offer(), offerFirst(), offerLast() in Java
- LinkedList add() method in Java
- LinkedList addAll() method in Java
- LinkedList descendingIterator in Java with Examples
- LinkedList listiterator() method in Java
- LinkedList remove() Method in Java
- How to Implement Generic LinkedList in java
- LinkedList implementation in JavaScript
- Add 1 To Number Represented As Linked List
- Add One To The Number
- Add two numbers represented by linked lists | Set 1
- Add two numbers represented by linked lists | Set 2
- Adding two polynomials using Linked List
- An interesting method to print the reverse of a linked list.
- Arrange consonants and vowels nodes in a linked list
- Binary search on Linked List
- Bubble Sort for Linked List by Swapping Nodes
- C Program For Merge Sort For Linked Lists
- C program for performing Bubble sort on Linked List
- C program to Reverse a Linked List
- Check if a linked list of strings forms a palindrome
- Check If Linked List Is Palindrome
- Check whether the length of given linked list is Even or Odd
- Clone a linked list with next and random pointer in O(1) space
- Compare two strings represented as linked lists
- Construct a Complete Binary Tree from its Linked List Representation
- Construct a linked list from a 2D matrix (Iterative approach)
- Construct a Maximum Sum Linked List out of two Sorted Linked Lists having some common nodes
- Convert a given Binary Tree to Doubly Linked List
- Convert a given Binary Tree To Doubly Linked List | Set 3
- Convert A String To A Singly Linked List
- Count duplicates in a Linked List
- Count pairs from two linked lists whose sum is equal to a given value
- Count rotations in a sorted and rotated linked list
- Create a Linked List From a Given Array
- Decimal Equivalent of Binary Linked List
- Delete a node at a given position
- Delete Alternate Nodes Of A Linked List
- Delete Kth Node From The End
- Delete middle of linked list
- Delete N nodes after M nodes of a Linked List
- Delete Nodes From Linked List
- Delete nodes that have a greater value on the right side
- Delete the last occurrence of an item from the linked list
- Delete the middle of a Linked List
- Detect and Remove the loop in a linked list
- Find a triplet from three linked lists with a sum equal to a given number
- Find Length Of A Linked List Iterative And Recursive
- Find modular node in a linked list
- Find pair for given sum in a sorted singly linked without extra space
- Find pair sum
- Find the first node of the loop in a Linked List
- Find the fractional (or n/k – th) node in linked list
- Find The Length of the Loop In The Linked List
- Find the smallest and largest elements in a singly linked list
- Find the sum of last n nodes of the given Linked List
- Flatten a multi-level linked list (Depth wise)
- Flatten Binary Tree To Linked List
- Function to delete a Linked list
- Given a Linked List move the last element to the front
- Given a linked list of line segments, remove middle points
- Given a Linked List which is sorted, how to insert in a sorted way
- Given only a pointer to a node to be deleted in a singly linked list, how do you delete it?
- How to Convert all LinkedHashMap Values to a List in Java
- How to get a Sublist of LinkedList in Java
- How to Get a Value from LinkedHashMap by Index in Java
- How To Iterate LinkedList in Java
- How To Write C Functions That Modify The Head Pointer Of A Linked List
- How to write C functions that modify the head pointer of a Linked List?
- Identical Linked Lists
- Implement a stack using a singly linked list
- Insert a nodeÂ
- Insert a node after the n-th node from the end
- Insert a node at a specific position in a linked list
- Insert a node into the middle of the linked list
- Insertion Sort for Singly Linked List
- Intersection point of two linked lists
- Iterative Selection Sort For Linked List
- Iteratively Reverse a Linked List Using Only 2 Pointers
- Java Program to Reverse a linked list
- Java Program to search an element in a Linked List
- Length of longest palindrome list in a linked list using O(1) extra space
- Linked List Deleting Node
- LinkedList removeFirst method in Java
- List Reduction
- Make loop at K-Th position in the Linked List
- Make middle node the head in a linked list
- Merge a Linked List into another Linked List at alternate positions
- Merge k sorted linked list
- Merge K sorted linked lists | Set 1
- Merge k sorted linked lists | Set 2 (Using Min Heap)
- Merge Sort on a Singly Linked List
- Merge two sorted linked lists
- Merge two sorted linked lists (in-place)
- Merge two sorted linked lists such that the merged list is in reverse order
- Merge Two Unsorted Linked Lists To Get A Sorted List
- Modify contents of Linked List
- Move all occurrences of an element to end in a linked list
- Move the first element to the end of the given list
- Multiplication of two polynomials using Linked List
- Multiply Linked Lists
- Multiply two numbers represented by Linked Lists
- Palindrome List
- Partitioning a linked list around a given value and If we don’t care about making the elements of the list “stable”
- Partitioning a linked list around a given value and keeping the original order
- Point arbit pointer to greatest value right-side node in a linked list
- Practice problem for linked list and Recursion
- Print Nodes Of Linked List At Given indexes
- Print Reverse Of A Linked List Without Actually Reversing it
- Print the reverse of a linked list without extra space and modifications
- Priority Queue using a Linked List
- Program to convert ArrayList to LinkedList in Java
- Program To Reverse A Linked List Using Stack
- Python Program to find the middle of a linked list using only one traversal
- Python Program to Reverse a linked list
- QuickSort on Singly Linked List
- Rearrange a given Linked List in-place
- Rearrange a linked list such that all even and odd positioned nodes are together
- Rearrange the given linked list such that it consists of alternating minimum-maximum elements
- Recursive selection sort for singly linked list | Swapping node links
- Recursively reversing a Linked List – A simple implementation
- Remove all occurrences of duplicates from a sorted linked list
- Remove all occurrences of duplicates from a sorted Linked List
- Remove duplicates from a sorted linked list
- Remove duplicates from an unsorted linked list
- Remove every Kth node of the Linked list
- Remove Nth Node From End Of The Linked List
- Remove the last node of a linked list
- Reorder list
- Reverse A Sublist Of Linked List
- Reverse Linked List
- Rotate a Linked List
- Run Length Decoding in Linked List
- Search an element in a Linked List (Iterative and Recursive)
- Segregate even and odd nodes in a Linked List
- Sort a Linked List of 0s, 1s and 2s
- Sort a linked list of 0s, 1s, and 2s by changing links
- Sort a linked list that is sorted alternating ascending and descending orders
- Squareroot(n)-th node in a Linked List
- Student Record Management System Using Linked List
- Sublist Search (Search a linked list in another list)
- Subtract two numbers represented as Linked Lists
- Swap Kth node from beginning with Kth node from end in the given Linked List
- Swap nodes in a linked list without swapping data
- The intersection of two Sorted Linked Lists
- The most efficient way to Pairwise swap elements of a given linked list
- Union and Intersection of two Linked Lists
- Union intersection Two Linked Lists Using Merge Sort
- Write a C function to print the middle of a linked list
- Can we reverse a linked list in less than O(n)?
- Implementing Iterator pattern of a single linked list